mirror of
https://github.com/libretro/dolphin
synced 2024-09-19 12:05:10 -04:00
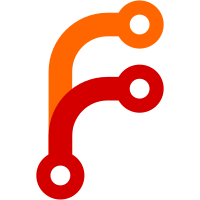
Ensures that the destruction logic is kept local to the translation unit (making it nicer when it comes to forward declaring non-trivial types). It also doesn't really do much to define it in the header.
38 lines
839 B
C++
38 lines
839 B
C++
// Copyright 2019 Dolphin Emulator Project
|
|
// Licensed under GPLv2+
|
|
// Refer to the license.txt file included.
|
|
|
|
#pragma once
|
|
|
|
#include <array>
|
|
#include <deque>
|
|
#include <functional>
|
|
#include <memory>
|
|
#include <string>
|
|
#include <utility>
|
|
|
|
class NetPlayChatUI
|
|
{
|
|
public:
|
|
explicit NetPlayChatUI(std::function<void(const std::string&)> callback);
|
|
~NetPlayChatUI();
|
|
|
|
using Color = std::array<float, 3>;
|
|
|
|
void Display();
|
|
void AppendChat(std::string message, Color color);
|
|
void SendMessage();
|
|
void Activate();
|
|
|
|
private:
|
|
char m_message_buf[256] = {};
|
|
bool m_scroll_to_bottom = false;
|
|
bool m_activate = false;
|
|
bool m_is_scrolled_to_bottom = true;
|
|
|
|
std::deque<std::pair<std::string, Color>> m_messages;
|
|
std::function<void(const std::string&)> m_message_callback;
|
|
};
|
|
|
|
extern std::unique_ptr<NetPlayChatUI> g_netplay_chat_ui;
|