mirror of
https://github.com/libretro/dolphin
synced 2024-11-09 06:53:01 -05:00
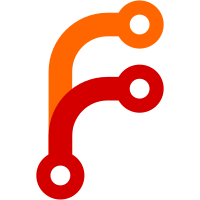
It is my opinion that nobody should use NKit disc images without being aware of the drawbacks of them. Since it seems like almost nobody who is using NKit disc images knows what NKit is (hmm, now how could that have happened...?), I am adding a warning to Dolphin so that you can't run NKit disc images without finding out about the drawbacks. In case someone really does want to use NKit disc images, the warning has a "Don't show this again" option. Unfortunately, I can't retroactively add the warning where it's most needed: in Dolphin 5.0, which does not support Wii NKit disc images.
32 lines
1 KiB
C++
32 lines
1 KiB
C++
// Copyright 2020 Dolphin Emulator Project
|
|
// Licensed under GPLv2+
|
|
// Refer to the license.txt file included.
|
|
|
|
#pragma once
|
|
|
|
#include <optional>
|
|
#include <string>
|
|
|
|
#include "Common/CommonTypes.h"
|
|
#include "DiscIO/Volume.h"
|
|
|
|
namespace DiscIO
|
|
{
|
|
class VolumeDisc : public Volume
|
|
{
|
|
public:
|
|
std::string GetGameID(const Partition& partition = PARTITION_NONE) const override;
|
|
Country GetCountry(const Partition& partition = PARTITION_NONE) const override;
|
|
std::string GetMakerID(const Partition& partition = PARTITION_NONE) const override;
|
|
std::optional<u16> GetRevision(const Partition& partition = PARTITION_NONE) const override;
|
|
std::string GetInternalName(const Partition& partition = PARTITION_NONE) const override;
|
|
std::string GetApploaderDate(const Partition& partition) const override;
|
|
std::optional<u8> GetDiscNumber(const Partition& partition = PARTITION_NONE) const override;
|
|
bool IsNKit() const override;
|
|
|
|
protected:
|
|
Region RegionCodeToRegion(std::optional<u32> region_code) const;
|
|
};
|
|
|
|
} // namespace DiscIO
|