mirror of
https://github.com/libretro/dolphin
synced 2024-11-04 20:43:51 -05:00
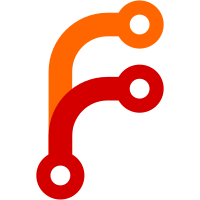
So far in all our uses of ScopeGuard, the type of the callable is usually just a lambda or a function pointer, so there is no need to rely on std::function's type erasure. While the cost of using std::function is probably negligible, it still causes some unnecessary overhead that can be avoided by making ScopeGuard a templated class. Thanks to class template argument deduction in C++17 most existing usages do not even need to be changed. See https://godbolt.org/z/KcoPni for a comparison between a ScopeGuard that uses std::function and one that doesn't
44 lines
801 B
C++
44 lines
801 B
C++
// Copyright 2015 Dolphin Emulator Project
|
|
// Licensed under GPLv2+
|
|
// Refer to the license.txt file included.
|
|
|
|
#pragma once
|
|
|
|
#include <functional>
|
|
|
|
namespace Common
|
|
{
|
|
class ScopeGuard final
|
|
{
|
|
public:
|
|
template <class Callable>
|
|
ScopeGuard(Callable&& finalizer) : m_finalizer(std::forward<Callable>(finalizer))
|
|
{
|
|
}
|
|
|
|
ScopeGuard(ScopeGuard&& other) : m_finalizer(std::move(other.m_finalizer))
|
|
{
|
|
other.m_finalizer = nullptr;
|
|
}
|
|
|
|
~ScopeGuard() { Exit(); }
|
|
void Dismiss() { m_finalizer = nullptr; }
|
|
void Exit()
|
|
{
|
|
if (m_finalizer)
|
|
{
|
|
m_finalizer(); // must not throw
|
|
m_finalizer = nullptr;
|
|
}
|
|
}
|
|
|
|
ScopeGuard(const ScopeGuard&) = delete;
|
|
|
|
void operator=(const ScopeGuard&) = delete;
|
|
|
|
private:
|
|
std::function<void()> m_finalizer;
|
|
};
|
|
|
|
} // Namespace Common
|