mirror of
https://github.com/libretro/dolphin
synced 2024-11-04 20:43:51 -05:00
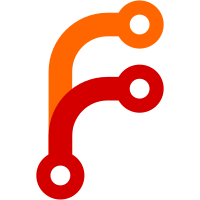
In cases where we just want a random value for a primitive arithmetic type, we can wrap this in a template to allow convenient direct assignment instead of keeping declaration and initialization separate (making it more difficult to use values uninitialized). This also allows the use of Common::Random with functions such as std::generate, making it more flexible in how random values can be generated.
27 lines
659 B
C++
27 lines
659 B
C++
// Copyright 2018 Dolphin Emulator Project
|
|
// Licensed under GPLv2+
|
|
// Refer to the license.txt file included.
|
|
|
|
#pragma once
|
|
|
|
#include <cstddef>
|
|
#include <type_traits>
|
|
|
|
#include "Common/CommonTypes.h"
|
|
|
|
namespace Common::Random
|
|
{
|
|
/// Fill `buffer` with random bytes using a cryptographically secure pseudo-random number generator.
|
|
void Generate(void* buffer, std::size_t size);
|
|
|
|
/// Generates a random value of arithmetic type `T`
|
|
template <typename T>
|
|
T GenerateValue()
|
|
{
|
|
static_assert(std::is_arithmetic<T>(), "T must be an arithmetic type in GenerateValue.");
|
|
T value;
|
|
Generate(&value, sizeof(value));
|
|
return value;
|
|
}
|
|
} // namespace Common::Random
|