mirror of
https://github.com/libretro/dolphin
synced 2024-11-04 20:43:51 -05:00
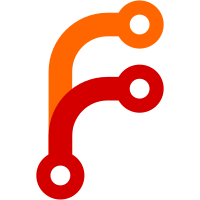
This function only ever reads the contents of the string in a non-owning manner, so we can change the parameter over to being a string view.
33 lines
589 B
C++
33 lines
589 B
C++
// Copyright 2014 Dolphin Emulator Project
|
|
// Licensed under GPLv2+
|
|
// Refer to the license.txt file included.
|
|
|
|
#pragma once
|
|
|
|
#include <array>
|
|
#include <optional>
|
|
#include <string>
|
|
#include <string_view>
|
|
|
|
#include "Common/CommonTypes.h"
|
|
|
|
namespace Common
|
|
{
|
|
enum class MACConsumer
|
|
{
|
|
BBA,
|
|
IOS
|
|
};
|
|
|
|
enum
|
|
{
|
|
MAC_ADDRESS_SIZE = 6
|
|
};
|
|
|
|
using MACAddress = std::array<u8, MAC_ADDRESS_SIZE>;
|
|
|
|
MACAddress GenerateMacAddress(MACConsumer type);
|
|
std::string MacAddressToString(const MACAddress& mac);
|
|
std::optional<MACAddress> StringToMacAddress(std::string_view mac_string);
|
|
} // namespace Common
|