mirror of
https://github.com/dolphin-emu/dolphin
synced 2024-11-04 20:43:44 -05:00
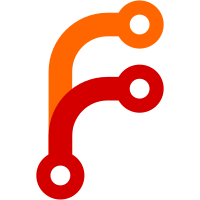
SPDX standardizes how source code conveys its copyright and licensing information. See https://spdx.github.io/spdx-spec/1-rationale/ . SPDX tags are adopted in many large projects, including things like the Linux kernel.
51 lines
1.2 KiB
C++
51 lines
1.2 KiB
C++
// Copyright 2018 Dolphin Emulator Project
|
|
// SPDX-License-Identifier: GPL-2.0-or-later
|
|
|
|
#pragma once
|
|
|
|
#include <list>
|
|
#include <mutex>
|
|
#include <thread>
|
|
|
|
#include <QObject>
|
|
|
|
#include "Common/Event.h"
|
|
#include "Common/Flag.h"
|
|
|
|
#include "UICommon/DiscordPresence.h"
|
|
|
|
class DiscordJoinRequestDialog;
|
|
|
|
class DiscordHandler : public QObject, public Discord::Handler
|
|
{
|
|
Q_OBJECT
|
|
#ifdef USE_DISCORD_PRESENCE
|
|
public:
|
|
explicit DiscordHandler(QWidget* parent);
|
|
~DiscordHandler();
|
|
|
|
void Start();
|
|
void Stop();
|
|
void DiscordJoin() override;
|
|
void DiscordJoinRequest(const char* id, const std::string& discord_tag,
|
|
const char* avatar) override;
|
|
void ShowNewJoinRequest(const std::string& id, const std::string& discord_tag,
|
|
const std::string& avatar);
|
|
#endif
|
|
|
|
signals:
|
|
void Join();
|
|
void JoinRequest(const std::string id, const std::string discord_tag, const std::string avatar);
|
|
|
|
#ifdef USE_DISCORD_PRESENCE
|
|
private:
|
|
void Run();
|
|
QWidget* m_parent;
|
|
Common::Flag m_stop_requested;
|
|
Common::Event m_wakeup_event;
|
|
std::thread m_thread;
|
|
std::list<DiscordJoinRequestDialog> m_request_dialogs;
|
|
std::mutex m_request_dialogs_mutex;
|
|
#endif
|
|
};
|